As programmers and software engineers, we all appreciate simplicity and clarity in our code. That’s why it’s essential to have a lean CSS system that is easy to maintain and update. In this article, we’ll cover how to create a lean 8-Grid CSS system that uses an 8-grid system for spacing, margin, and line heights and includes flexbox for flexible layouts. We’ll also look at how to organize CSS into separate files for different page elements such as buttons and forms.
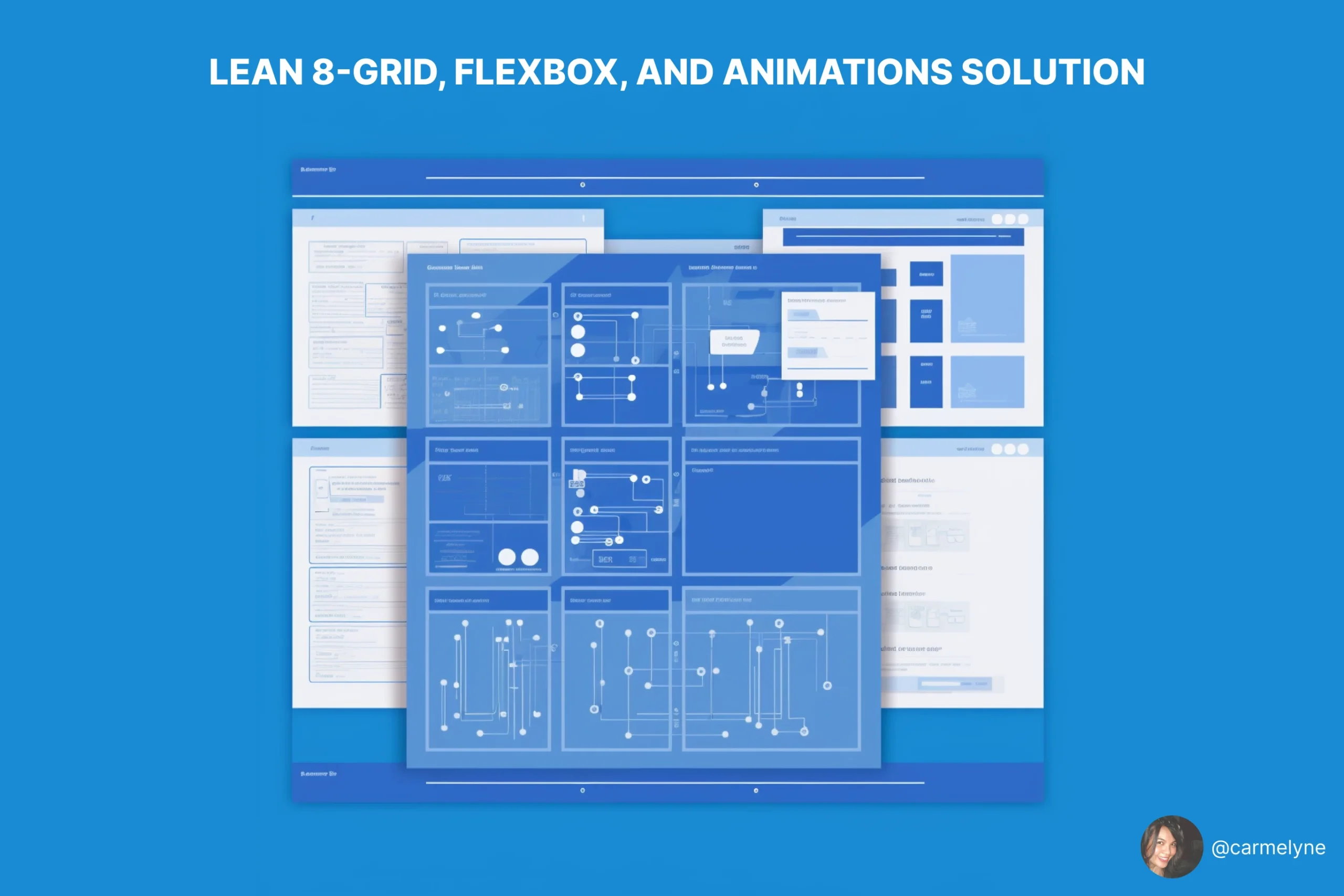
Rem vs Pixels
Before we dive into the 8-grid system and flexbox, let’s first talk about the advantages of using rem
units over pixels
for font sizing and spacing in CSS.
rem
units provide greater flexibility in responsiveness and are easier to maintain. Unlike pixels
, rem
units are relative to the root font size, which means that they adjust automatically when the user changes the default font size in their browser. For example, if you set the root font size to 16px
and use 1rem
for your font size, it will be equivalent to 16px
. If the user changes their browser’s default font size to 20px
, 1rem
will now be equivalent to 20px
. This makes rem
units more accessible and user-friendly.
To convert px
values to rem
units, you need to use a base font size of 16px
. For example, if you want to set a margin of 40px
, you can convert it to 2.5rem
by dividing 40
by 16
.
The 8-Grid CSS System
The 8-grid system is a simple way to maintain consistency in your CSS by dividing your page into eight equal vertical units. Each unit represents a specific value that you can use for spacing, margin, and line heights. The 8-grid system works well with rem
units because it provides a predictable and consistent scale for your page layout.
Here’s an example of how to set up the 8-grid system in your CSS:
.mt-1 {
margin-top: 0.125rem;
}
.mt-2 {
margin-top: 0.25rem;
}
.mt-3 {
margin-top: 0.375rem;
}
.mt-4 {
margin-top: 0.5rem;
}
.mt-5 {
margin-top: 0.625rem;
}
.mt-6 {
margin-top: 0.75rem;
}
.mt-7 {
margin-top: 0.875rem;
}
.mt-8 {
margin-top: 1rem;
}
In this example, we’re using classes to set the margin-top
property to different values based on the 8-grid system. You can use these classes to maintain consistency in your page layout and make it easier to update in the future.
Lean CSS
Now that we have the 8-grid system set up, let’s create a lean CSS system that includes a base CSS file with core styles, a flexbox CSS file with styles for flexible layout, and an animations CSS file with basic animation styles.
Base CSS File
The base CSS file includes core styles for typography, color, and spacing. Here’s an example of what it might look like:
/* Typography */
h1 {
font-size: 2rem;
font-weight: bold;
margin-bottom: 1rem;
}
p {
font-size: 1rem;
line-height: 1.5rem;
margin-bottom: 1.5rem;
}
/* Color */
body {
color: #333;
background-color: #fff;
}
/* Spacing */
.container {
max-width: 1200px;
margin: 0 auto;
padding: 0 1rem;
}
In this example, we’re setting the font-size
and line-height
for h1
and p
elements using rem
units based on the 8-grid system. We’re also setting the color and background-color for the body
element and the maximum width and padding for the .container
class.
Flexbox CSS File
The flexbox CSS file includes styles for flexible layout using flexbox. Here’s an example of what it might look like:
/* Flexbox */
.flex {
display: flex;
}
.flex-column {
flex-direction: column;
}
.flex-row {
flex-direction: row;
}
.items-center {
align-items: center;
}
.justify-center {
justify-content: center;
}
In this example, we’re setting up some basic flexbox classes that can be used to create flexible layouts. The .flex
class sets the display
property to flex
, which turns an element into a flex container. The .flex-column
and .flex-row
classes set the flex-direction
property to column
and row
, respectively. The .items-center
class aligns the items in the center vertically, and the .justify-center
class aligns the items in the center horizontally.
Animations CSS File
The animations CSS file includes basic animation styles that can be used to enhance the user experience. Here’s an example of what it might look like:
/* Animations */
.fade-in {
opacity: 0;
animation: fadeIn ease-in-out 1s;
}
@keyframes fadeIn {
0% {
opacity: 0;
}
100% {
opacity: 1;
}
}
.slide-in {
transform: translateX(-100%);
animation: slideIn ease-in-out 1s;
}
@keyframes slideIn {
0% {
transform: translateX(-100%);
}
100% {
transform: translateX(0%);
}
}
.scale-in {
transform: scale(0);
animation: scaleIn ease-in-out 1s;
}
@keyframes scaleIn {
0% {
transform: scale(0);
}
100% {
transform: scale(1);
}
}
In this example, we’re setting up some basic animation classes that can be used to fade, slide, and scale elements. The .fade-in
class sets the opacity
property to 0
and applies a fadeIn
animation that gradually increases the opacity from 0
to 1
over a duration of 1s
. The .slide-in
class translates the element 100% to the left and applies a slideIn
animation that gradually moves the element back to its original position over a duration of 1s
. The .scale-in
class scales the element to 0
and applies a scaleIn
animation that gradually increases the scale to 1
over a duration of 1s
.
Organizing CSS Files
Now that we have our base, flexbox, and animations CSS files, we can organize them into separate files for different page elements such as buttons and forms. This helps to keep the CSS lean and modular. Here’s an example of what a button CSS file might look like:
/* Button Styles */
.button {
background-color: #3b82f6;
color: #fff;
border-radius: 0.25rem;
padding: 0.5rem 1rem;
font-size: 1rem;
line-height: 1.5rem;
cursor: pointer;
transition: all 0.3s ease-in-out;
}
.button:hover {
background-color: #1f6feb;
}
In this example, we’re setting up a basic style for a button that includes a background color, border-radius, padding, font-size, line-height, and cursor. We’re also applying a hover effect that changes the background color when the user hovers over the button.
Here’s an example of what a form CSS file might look like:
/* Form Styles */
.input {
font-size: 1rem;
line-height: 1.5rem;
padding: 0.5rem;
border: 1px solid #ccc;
border-radius: 0.25rem;
}
.input:focus {
outline: none;
border-color: #3b82f6;
}
.input-error {
border-color: #dc2626;
}
.input-error:focus {
border-color: #dc2626;
}
In this example, we’re setting up styles for form inputs that include a font-size, line-height, padding, border, and border-radius. We’re also applying styles for when the input is in focus or has an error.
In this article, we covered how to create a lean CSS system that uses an 8-grid system for spacing, margin, and line heights and includes flexbox for flexible layouts. We also looked at how to organize CSS into separate files for different page elements such as buttons and forms. By using this lean CSS system, you can create a simple and easy-to-maintain CSS codebase that will help you build great-looking websites with ease.
Remember, it’s always a good idea to keep your CSS codebase as lean as possible to improve page load times and make it easier to maintain in the long run. Hopefully, this article has given you some useful insights and ideas to help you create a more efficient CSS system.